Starting With Rails
In my last post I walked you through installing Ruby on your machine, be it a Mac, Windows, or Linux computer. If you remember, I planned to help my developer friend set up Rails on his machine. So with Ruby set up, you’re ready to install Rails. Let’s get started.
Windows Specifics
Like last time, I’ll start with Windows. If you followed along last time, you might have used the Rails Installer. If you did, excellent, you can skip ahead to the next section. If you went the Ruby Installer route, we’re going to need to install one thing before working with Rails. Out of the box, Rails uses SQLite as it’s default data store. SQLite is an easy to install, self-contained SQL database engine. It’s easy to use and supports up to 140 TB of data; Rails chose SQLite. Even if you decide to move to something else, like Postgres, you might find yourself using SQLite in your local test environment. Once you use something that is Postgres specific, you’ll then need to use it in all your environments, but that’s getting ahead of ourselves.
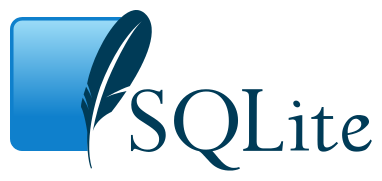
Head over to the SQLite Download Page, and grab the precompiled binary for Windows. There is a 32-bit and 64-bit version of SQLite; I’d match the version to your version of Windows, which is most likely 64-bit. Unpack the zip archive to a folder in your PATH, such as the C:\WINDOWS\system32
folder, or even the Ruby bin folder (typically C:\Ruby\bin
). That’s all there is to it.
RubyGems
Before we install Rails, I’d just like to talk a little about RubyGems. Gems (for short) are either written in Ruby or C, as I mentioned in the last post. When you issue a gem install
command, by default, the gem will be installed as well as documentation for the gem. Honestly, this is just a waste of local space. To change the default behavior, tell it to skip documentation, run the following command:
echo 'gem: --no-document' >> ~/.gemrc
That command will work on Mac and Linux, for Windows, where you don’t really have a “home directory,” the one-liner command for you is:
(echo install: --no-document && echo update: --no-document) >> c:\ProgramData\gemrc
Note that by installing it in
ProgramData
it applies to all users.
With the .gemrc
(or gemrc
in Windows) in place, you can install any gem and know that it will skip installing documentation for every gem you install.
Installing Rails
This section is going to be very short. Rails is just a collection of gems.
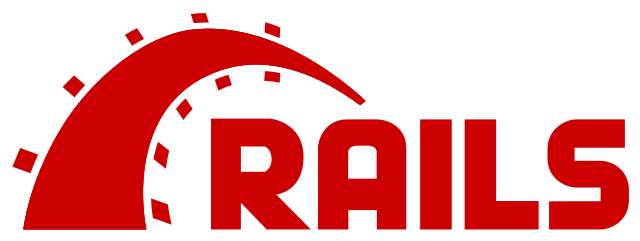
All we need to do is run the command to install the latest version of Rails:
gem install rails
That’s it! Gems are a snap to install.
Running an Existing Rails Application
Installing Rails is super easy, but what if you want to run an existing Rails application that you download from say GitHub. You don’t have to install Rails directly. As long as the project has a Gemfile
, you can use a gem called Bundler. The Gemfile
in the root of the project specifies it’s dependencies, and in a Rails project you’re going to find an entry like gem 'rails', '5.1.5'
. This line tells Bundler to install Rails v5.1.5. Because of this, we don’t have to install Rails separately; we can just use Bundler. But before we use Bundler, we need to install it, note we’ll only need to do this once:
gem install bundler
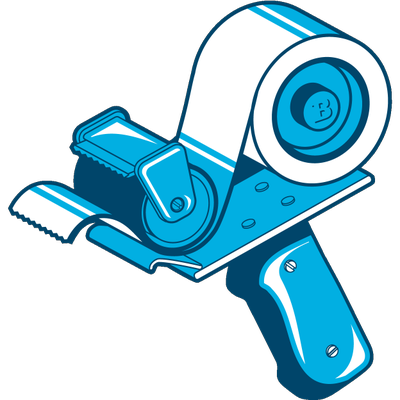
Now that we have Bundler, we can navigate to the project’s root folder (where the GemFile
is and run the simple command:
bundle install
Bundler will kick into action and install all the dependencies required by the Gemfile
.
Want to work on different Rails project? Just bundle install
in its root directory, that’s all there is to it.
A Simple “App”
Congrats, you’ve reached the end of the tutorial. You now know how to install Rails, easy, isn’t it? We learned a little more about RubyGems and then discovered Bundler.
If you want to test your Rails install out, we can create a little, super-simple application in just a few commands. Now, this is nowhere near a production app, but it will get you to verify everything works. I’m going to use the scaffold
generator that Rails has to whip up something quickly, but you’ll see that the scaffold
generator generates a lot of things we don’t want. With that said, open up a Terminal session and type the following:
rails new notepad
cd notepad
rails g scaffold note title content:text
rails db:migrate
rails s
These five commands will do the following:
- Create a new application called notepad and run
bundle install
-rails new notepad
- Change into the newly created notepad directory -
cd notepad
- Create scaffolding -
rails g scaffold note title content:text
- This will generate a database migration file
- A
Note
model withtitle
as astring
andcontent
as atext
field - A
NotesController
which will have actions defined on it - A
/notes
route that will handleGET
,POST
,PUT/PATCH
, andDELETE
- Tests for your model, route, controller
- A
NotesHelper
- Views for your controller actions, both ERB and JBuilder files
- A CoffeeScript file
- Finally two SCSS files, one for scaffolds and one specifically for notes.
- Create the SQLite database and run the migration file -
rails db:migrate
- Finally, run the development server
Now browse to http://localhost:3000/notes
, and you’ll see you can add, update, create, and delete notes. A straightforward and simplistic app. Oh, by the way, the rails g
command is a shortcut for rails generate
and rails s
is short for rails server
. You can use either command, but I prefer less typing with the shortcuts. Below you can see the result in action.
Wrap Up
I hope you enjoyed this post. We just scratched the surface of Rails, but we installed it and made sure it worked. We utilized the scaffold generator, and I’m sure you realized that the scaffolding generates a lot of files that we don’t need. We’ll see in another post other Rails generators so we can pick and choose what we want. As always, please let us know what you think, either leave a comment or Contact Us.