Ember Octane + ESLint + Prettier
Introduction
I started a brand new Ember Octane project today. The first thing I wanted to do was set up prettier in my Ember app. I started using prettier for a client, and I adopted the practice myself. If you aren’t familiar with prettier, it’s a library that formats your code in an opinionated way. Not everyone will like the defaults, but prettier is very configurable (which I will show you).
Note these steps can be used for any JavaScript project. I’ll show the changes to a new Ember Octane project, but the NPM libraries will work in other projects as well.
Overview
If you aren’t familiar with prettier, take the example below (from the README on GitHub).
Input
// Given this line of code...
foo(reallyLongArg(), omgSoManyParameters(), IShouldRefactorThis(), isThereSeriouslyAnotherOne());
Output
// You'll end up with something like this...
foo(
reallyLongArg(),
omgSoManyParameters(),
IShouldRefactorThis(),
isThereSeriouslyAnotherOne()
);
As you can see it really cleans up your code.
Install
You are going to need a few node packages:
npm install --save-dev eslint-plugin-prettier
npm install --save-dev --save-exact prettier
npm install --save-dev eslint-config-prettier
Let’s look at these packages.
- The first,
eslint-plugin-prettier
, allows prettier and eslint to work together. - The second,
prettier
, is the prettier library. - Finally,
eslint-config-prettier
turns off eslint rules that conflict with prettier.
Configuration
Once you install these, you’re going to have to modify your .eslintrc.js
file.
Your extends
section should look like this:
extends: [
'plugin:prettier/recommended',
'eslint:recommended',
'plugin:ember/recommended',
],
The new item there is: 'plugin:prettier/recommended'
,
This does three things:
- Enables
eslint-plugin-prettier
. - Sets the
prettier/prettier
rule to"error"
. - Extends the
eslint-config-prettier configuration
.
Now you can customize prettier with a .prettierrc
file. This can be YAML or JSON. I’ve opted for JSON, and the following is my config:
{
"printWidth": 80,
"tabWidth": 2,
"useTabs": false,
"semi": true,
"singleQuote": true,
"trailingComma": "all",
"bracketSpacing": true,
"arrowParens": "avoid",
"requirePragma": false,
"insertPragma": false,
"proseWrap": "preserve"
}
This config was generated by Michael Larson’s Generator. It’s an excellent tool for customizing prettier easily. I recommend that you use this to personalize prettier to your liking.
Running Prettier on Your Code
I have a new Ember Octane project that I started. There is one commit in the git history for the initial Ember Octane application. I wanted to start with a clean, pretty project. I executed prettier from the terminal to update everything in my project:
prettier --write '\*_/_.{js,css,html}'
This updates all the default files for a new Ember Octane project. You’ll end up modifying ten of Ember Octane’s default files. Also, in Figure 01 below, you’ll notice the changes to package.json
and package-lock.json
for the addition of the node libraries. Another file is .prettierrc
which holds our prettier configuration.
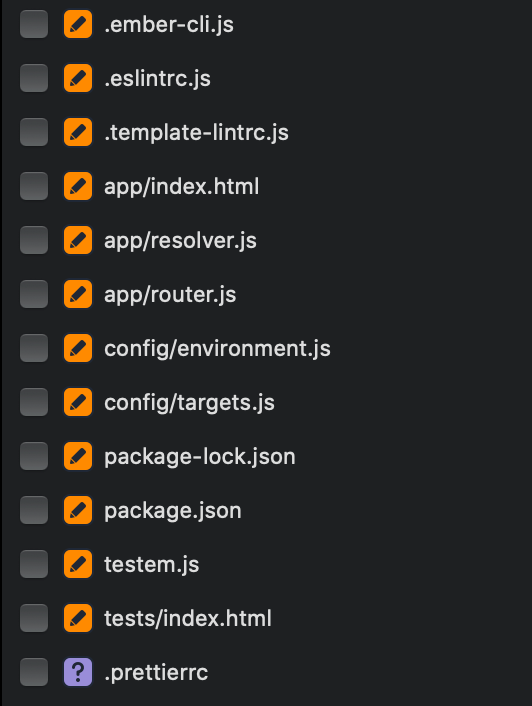
Editor Enhancements
To enhance our developer experience, we can use editor packages to make using prettier easier. I use Atom, and there is a prettier package that will automatically format your code on save (if you choose, Figure 02).
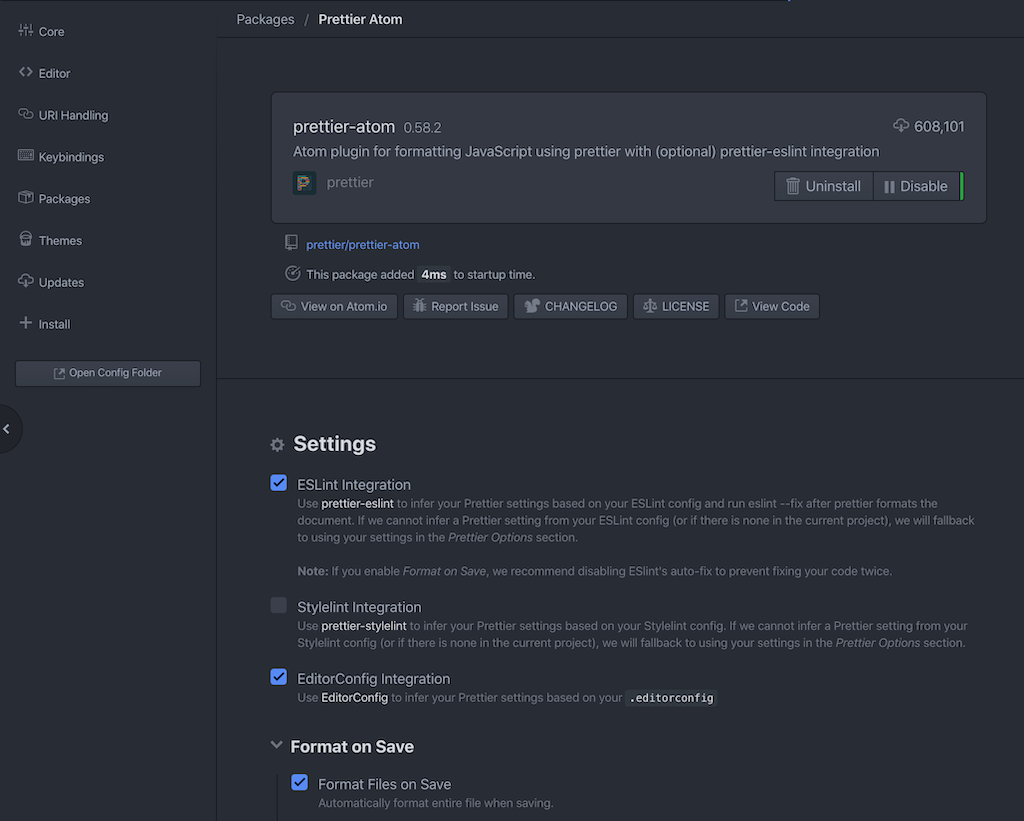
Note in Figure 02, there is the first option to use prettier with eslint, make sure you check that for your Ember Octane project.
Once installed, when you are on a JavaScript file, you’ll notice a prettier option in your status bar (Figure 03)

If, for some reason, you don’t want your file to be formatted, you can click on the green icon and disable it from updating on save. Of course, that sort of defeats the purpose of keeping your code “pretty.”
Conclusion
I like using it to format my code in a deterministic way. I don’t have to worry about other devs or even myself from creating messy code. If you want to take this to the next level, have prettier run when you commit to GitHub with an Action